OCaml Documentations as one-pagers, easy to keep useful commands in mind - OCamlPro/ocaml-cheat-sheets. ◆ Website ◆ (The listing sheet, as PDF, can be found here, or as a single column portrait, while below is an unruly html rendition. This reference sheet is built from a CheatSheets with Org-mode system. A course on functional programming at Cornell University.
The listing sheet, as PDF, can be foundhere,or as a single column portrait,while below is an unruly html rendition.
This reference sheet is built from aCheatSheets with Org-modesystem.
- Emacs Setup:HTML:
OCaml is a strict language;it is strongly typed where types are inferred.
I may write explicit type annotations below for demonstrationor clarity purposes.
Only when interacting with the top-level interpreter,commands must be terminated by ;;
.OCaml uses ;
as an expression separator —not a terminator!
My Emacs setup for OCaml can be found on this CheatSheet’s repo.
First, let’s get [Meta]OCaml.
Let’s set this up for Emacs.
Let’s obtain ocp-indent
—OCaml’s indentation tool that indents the same even ifco-workers use a different editor— and merlin which provides interactive feedbackincluding context-aware completion and jumping to definitions.
Now entering, say, List.
brings a pop-up completion menu for thecontents of the list module.
—In org-src blocks, you need to enter the ocaml mode, via C-c '
.
Operations on floats have a ‘.’ suffix.
A function is declared with the let
keyword—variables are functions of zero arguments.Function & varaible names must begin with a lowercase letter, and may use _ or '
.
- They cannot begin with capital letters or numbers, or contain dashes!
- Functions are like variables, but with arguments, so the same syntax applies.
Ocaml Cheat Sheet 2020
Here’s an example of a higher-order function & multiple local functions& an infix operator & an anonymous function & the main method isparametricly polymorphic.
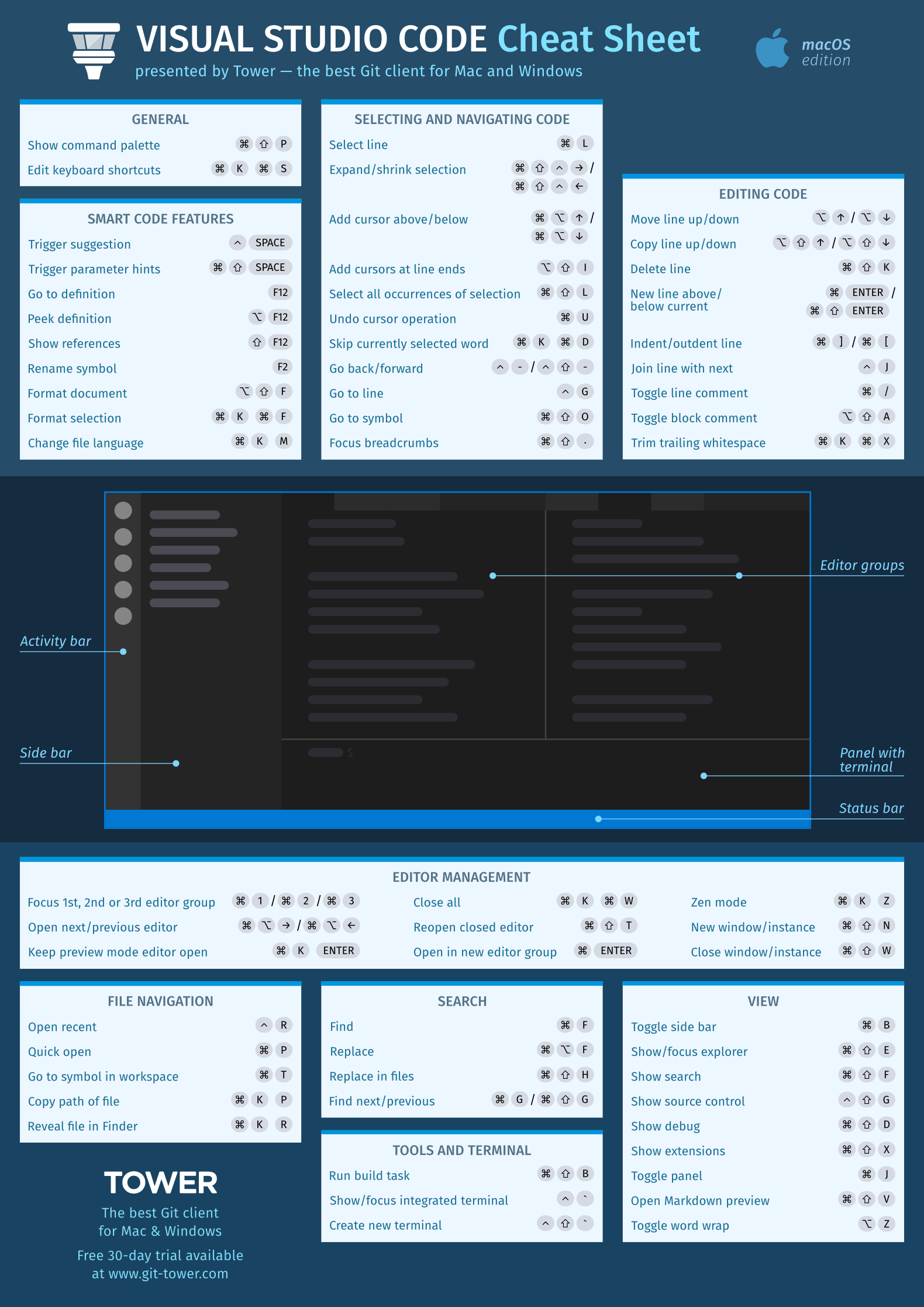
Inequality is expressed with <>
.
OCaml strings are not arrays, or lists, of characters as in C or Haskell.
Records: Products with named, rather than positional, components.
Variant types: A unified way to combine different types into a single type;each case is distinuighed by a capitalised tag.
The tags allow us to extract components of a variant valueas well as to case against values by inspecting their tags.This is pattern matching.
Note that we can give a pattern a name; above we mentioned p
,but did not use it.
Repeated & non-exhaustive patterns trigger a warning; e.g., remove the default case above.
You can pattern match on arrays too; e.g.,
[| x ; y ; z|] -> y
.
The above mechanisms apply to all variants —including tuples, lists, and options.
Tuples: Parentheses are optional, comma is the main operator.
columnbreak
Option: Expressing whether a value is present or not.
We may use begin/end or parentheses to groupexpressions together.
Remember: Single semicolon ;
is for sequencing whereas double ;;
is for termination.
OCaml programs don’t have a unique main
function as in C, instead the entire fileis evaluated sequentially at startup, which may contain arbitrary expressions not just functional declarations,and so in some sense the full codebase is one big main
function.
Zero-indexed Arrays: Indexing with .(i)
and update with <-
.
What is the type of update, <-
? A function that returns the unit type!—see myupdate
below.
Operations whose use produces a side-effect return the unit
type.This’ akin to the role played by void
in C.A function is a sequence of expressions; its return valueis the value of the final expression —all other expressionsare of unit type.
Record fields are immutable by default, but can be declared mutable.
References: Single mutable values; i.e., a record with a single mutable field named contents
.
Refs come with a handful of convenience methods; here’s their re-implementation:
Notice that ref
Dirt 2 for mac. is overloaded: As a type former and as a function forming values.
columnbreak
OCaml files not only store & manage code, but also correspond to (second-class) ‘modules’,which act as boundaries that divide a program into conceptual units.
At its simplest, a module is a collection of definitions that are storedwithin a namespace.
- Implementation details of a module can be hidden by using aninterface, module type, signature —all are aliases.
val
declarations specify values in a signature:val ⟪identifier⟫ : ⟪type⟫
.- A type is abstract if its name is exposed in the interface but its definition is not:
type t
.- It is conventional to use
t
for the name of such types.
- It is conventional to use
- Including the type definition in the interface makes the type concrete.
E.g., module names are derived, with capitalisation, automatically from file names.An interface for
myfile.ml
to constrain exposed interface is placed inmyfile.mli
.This is nearly how C header and implementation files work.
- Modules & signatures can be nested inside other modules.
Modules names always begin with a capital; their contents are accessed withdot notation. Here is the general syntax:
Without constructors type
creates aliases, if we want to treat a type in two differentways and have the compiler ensure we don’t mess-up, we produce single-constructor newtypes:
However, if the underlying type were the same, this repetition could be error-prone.Instead, we could use generative modules: Distinct types with the same underlying implementation.Being ‘generative’ is akin to the new
keyword in Java: Each use of the BasicImplementation
module below makes a new type.
Note that BasicImplemention
is just a namespace where, e.g., t
is an alias for string
.
Note that we could have placed the definitions of EssentiallyString
and newline BasicImplementaion
in-line for the Person
and Address
moduledeclarations —without syntactic alterations— but that would havedefeated our purpose of avoiding repetition.
Without the type annotation, we could accidentally forget to implementpart of the interface & OCaml would infer a different module type.Use the module type of
operator to see what was inferred.
Many equivalent ways to use module contents —the third is for small expressions.
Note the dot!
let qasim = Person.(from_string “jasim”)in (let open Person in qasim = jasim) , Person.(=) qasim jasim , Person.(from_string “jasim” = jasim) (* Rebind module name to a shorter name *) , let module P = Person in P.(qasim = jasim);;
While opening a module [type] affects the environment used to search for identifiers,including a module is a way of adding new identifiers to a module [type] proper—it is a copy-paste of constituents.For example, we could re-organise EssentialyString
into a hierarchy:
This allows us to form extensions to modules, akin to C#:To extend, say, the List
module with a new function f
so thatList.f
is valid, we make and open module List = struct let f = ⋯ include List
.If we wanted to override an existing f
, we put its definition after the include.
The interface would be sig val f : ⋯ include (module type of List) end
.
When we have multiple declarations of a type or we have type that is too abstract to beof use, expect to see:Error: This expression has type 𝓉 but an expression was expected of type M.t
; instead maybe use M.t with type t = 𝓉
, where:
We may now continue our EssentiallyString
hierarchy:
Warning!The order of constructor declarations for a concrete type variant must be thesame in the implementation as in the interface, likewise for record fields andfunction arguments.
Functors are, roughly speaking, functions from modules to modules.— There’s no “modules to module types” thing, instead we returna module that contains a module type ;-) —
roomFunctors provide the same abstraction features as functions do but alsoallow the implementation of one module to vary depending on another module.In contrast to functions, functors require explicit type annotation—otherwise how would we know what constituents the input module contains—and their arguments must be enclosed in parentheses.
In a sense, these are parameterised modules —as in Agda— and that is howthey may be treated syntactically in OCaml:
A functor may be applied to any module that satisfies the functor’s input interface:
Modules can contain types & values, but ordinary values can’t contain modules ormodule types. In order to define variables whose value is a module, or a functionthat takes a module as an argument, OCaml provides first-class modules which areordinary values that can be created from and converted back to regular modules.
A first-class module is created by packaging up a module with a signature thatit satisfies by using the module
keyword:
Dot notation only works for records and modules, so we access contents of afirst-class module by turning it into an ordinary module with val
:
Warning! The parentheses for these module, val
keywords are important!
Rule of thumb: Use the forms (module M : T)
and (val M : T)
always.
We can create ordinary functions which consume and create first-class modules.
columnbreak Apple wallpapers for mac.
Type variables are generally implicit, but we can treat them as abstract typesin a function body yet still not pass them in explicitly at use-sites.
One use is to connect the abstract types of a first-class module with other typeswe’re working with —by using constraint sharing.That is, we ‘unbundle’ the type from ‘inside’ the module to its ‘type’.This is a prime reason to use first-class modules!
Here’s an example where we approximate C#’s default
keyword:
Conversely, this allows us to ‘bundle’ up data to form a module!
Quasi-quote expressions with ‘brackets’ .< expr >.
to delay their executionand splice quoted items into such an expression with ‘escape’ .~expr.
Finally, code can be ‘run’ with Runcode.run expr
.
Warning! You must use the metaocaml
command rather than the ocaml
command.
The traditional ‘staging of the power function’ examplecan be found here —as a Jupyter Notebook.
For more, see A Gentle Introduction to Multi-stage Programming
- The deprecated
!.
is replaced withRuncode.run
. - A Haskell rendition can be found here.
columnbreak
- Learn x in y minutes, where x = OCaml
- Try OCaml, online
- Real World OCaml
- OCaml meta tutorial —including 99 Problems
- Unix system programming in OCaml
OCaml-Top is designed for beginners andstudents, and specially tailored for exercises, practicals and simpleprojects
Features
Syntax coloring
Ocaml Cheat Sheet Printable
Automatic, state-of-the-art code indentation
Naturally avoids most beginner's syntactic mistakes
Intuitive code execution
'Run' button runs just what you would expect: the expression under cursor, and what is above if needed
Automatic type display
Put your cursor on the name of a standard library function, and the type shows up in the status bar. Non-intrusive, but here just when you need it.
Fully compatible with Microsoft Windows XP, Vista, 7 and 8, OSX and Linux.
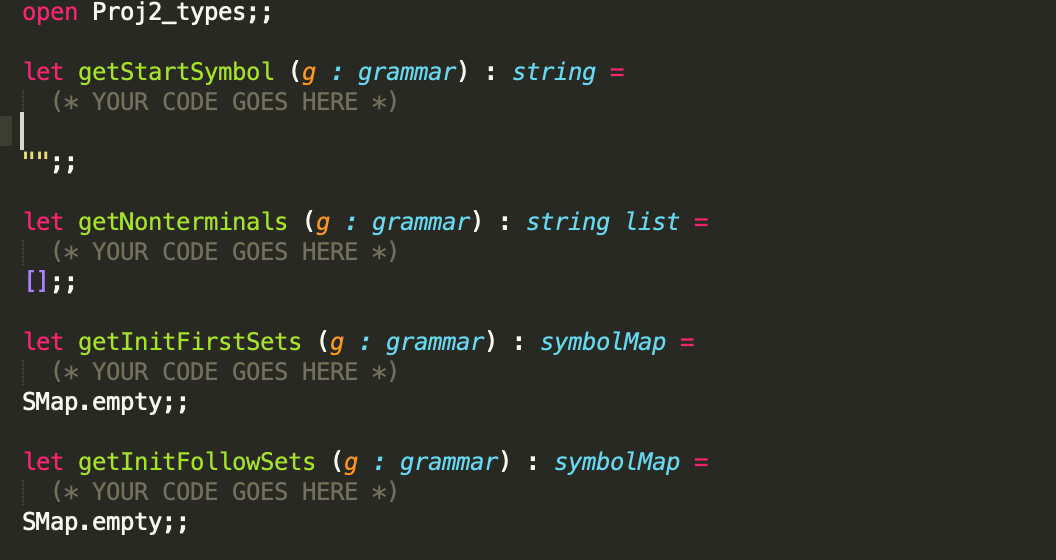
Documentation
Installation
On Linux or OSX, we recommend you use the OPAM package manager:
First install opam and some gtk dependancies. For example, with Ubuntu:
Then you need to install OCaml:

Avchd mts converter for mac. Finally install ocaml-top
On Microsoft Windows, download and run our binaryinstaller. You will also need a working installation of OCaml itself,for which werecommend theinstaller by Jonathan Protzenko. You need no extra options besides OCamlitself.
Usage
The left pane is the code editor, while the right pane contains the OCaml process that will execute the code.
The in the left margin of the editor is the evaluation mark, showing that the code has been successfully executed up to that point. When pressing the button (or Ctrl e), the expression under the cursor is sent to the OCaml pane, and the answer from OCaml is displayed there. In case of an error, the relevant part of the code in the editor is highlighted.
Since programs are usually written in order, the evaluation mark always progresses from top to bottom, and everything in-between the current mark position and the expression to run will be sent to OCaml first. If you happen to need execution of an expression directly, select it before pressing .
The button runs the whole program. In case you get confused, the button restarts a fresh OCaml, rewinds the evaluation mark, and clears the right pane. And in case you got yourself in a loop, or your program just takes too long, the button can interrupt the current computation.
Keyboard shortcuts
File management | |
---|---|
Ctrl n | Start with a new (blank) file |
Ctrl o | Load a file |
Ctrl s | Save the current file |
Edition | |
---|---|
Ctrl c | Copy |
Ctrl x | Cut |
Ctrl v | Paste |
Ctrl z | Undo |
Ctrl Shift z | Redo |
Execution | |
---|---|
Ctrl e | Execute the current expression |
Escape | Stop ongoing execution |
General | |
---|---|
Ctrl + | Zoom in |
Ctrl - | Zoom out |
F4 | Select font |
F5 | Switch colors |
F11 | Switch fullscreen |
Ctrl q | Quit |
Ocaml Cheat Sheet 2019
Resources
OCaml-Top on Github | Latest sources in the official GIT repository |
Version 1.1.2 | Download for Linux, OSX and Windows |
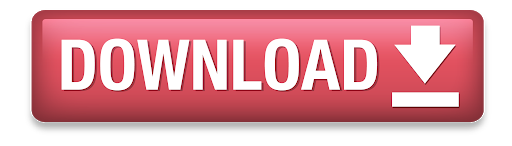